Challenges
Description
This challenge was picked from the r/dailyprogrammer subreddit. The challenge is to generate Julia set fractals from the complex number plane and produce an image as the output. This was a great chance to use Go as one of the bonus challenges is to make the solution run in parallel. This meant that using goroutines was an easy way to solve what could have been a much more difficult challenge.
I added a few options to the package so that the user can change the function that decides the Julia set (which also happened to be a bonus round). This is done by calling the
SetComplexFunction
function and supplying it with a function that has the signature of
func(complex128, complex128) complex128
. The first parameter is the current complex number on this iteration, and the second is the original complex number for the pixel value. This second parameter allows the generation of the basic Mandelbrot set. I've also added the option to change the complex number range, this allows me to zoom in or out of a particularly interesting set (I use this to view the whole Mandelbrot set).
Mandelbrot Set
Even though this package was mostly built for the creation of Julia set images, you can also display the Mandelbrot set and other complex fractals by using the
SetComplexFunction
. Mandelbrot is defined by the function $f_c(z) = z^2 + c$ where $c$ is the complex number and $z$ is the iteration. This is more explicit when represented recursively, $z_{n} = z^2_{n-1} + c$. The code snippet below shows how to draw the Mandelbrot set using this package.
julia.SetComplexFunction(func(c, orig complex128) complex128 {
return c*c + orig
})
julia.SetComplexRange(complex(-2.5, -1), complex(1, 1))
julia.CreateImage("image.png", 1920, 1080)
Zooming Into A Fractal
As previously mentioned you can zoom into a fractal by using the
SetComplexZoom
and
SetMaxIteration
functions. We can also alter the contrast of the image using the
SetContrast
function. This will help to show the patterns more clearly.
The snippet below shows how to zoom into the Mandelbrot set. For other interesting coordinates, you can look at http://www.cuug.ab.ca/dewara/mandelbrot/images.html.
julia.SetComplexFunction(func(c, orig complex128) complex128 {
return c*c + orig
})
julia.SetColorFunction(func(col uint8) *color.RGBA {
return &color.RGBA{col, col, col, 255}
})
julia.SetComplexZoom(complex(0.2549870375144766, -0.0005679790528465), 1.0e-13)
julia.SetContrastValue(50.0)
julia.SetMaxIteration(5000)
julia.CreateImage("image.png", 1920, 1080)
Feedback From A Friendly Redditor
After submitting my solution to the daily challenge, I received some great feedback from a more experienced Go programmer. One of this redditor's helpful suggestions was to re-evaluate my goroutines. This is because I could take advantage of pre-calculating some information, instead of having to calculate it for each pixel in the image. This was a huge speed increase for the project. Another useful tidbit was to use a named return variable. This is a really great way of making code more readable. While I had read about this in The Go Programming Language (Addison-Wesley) book, I could not truly appreciate it until now.
Screenshots:
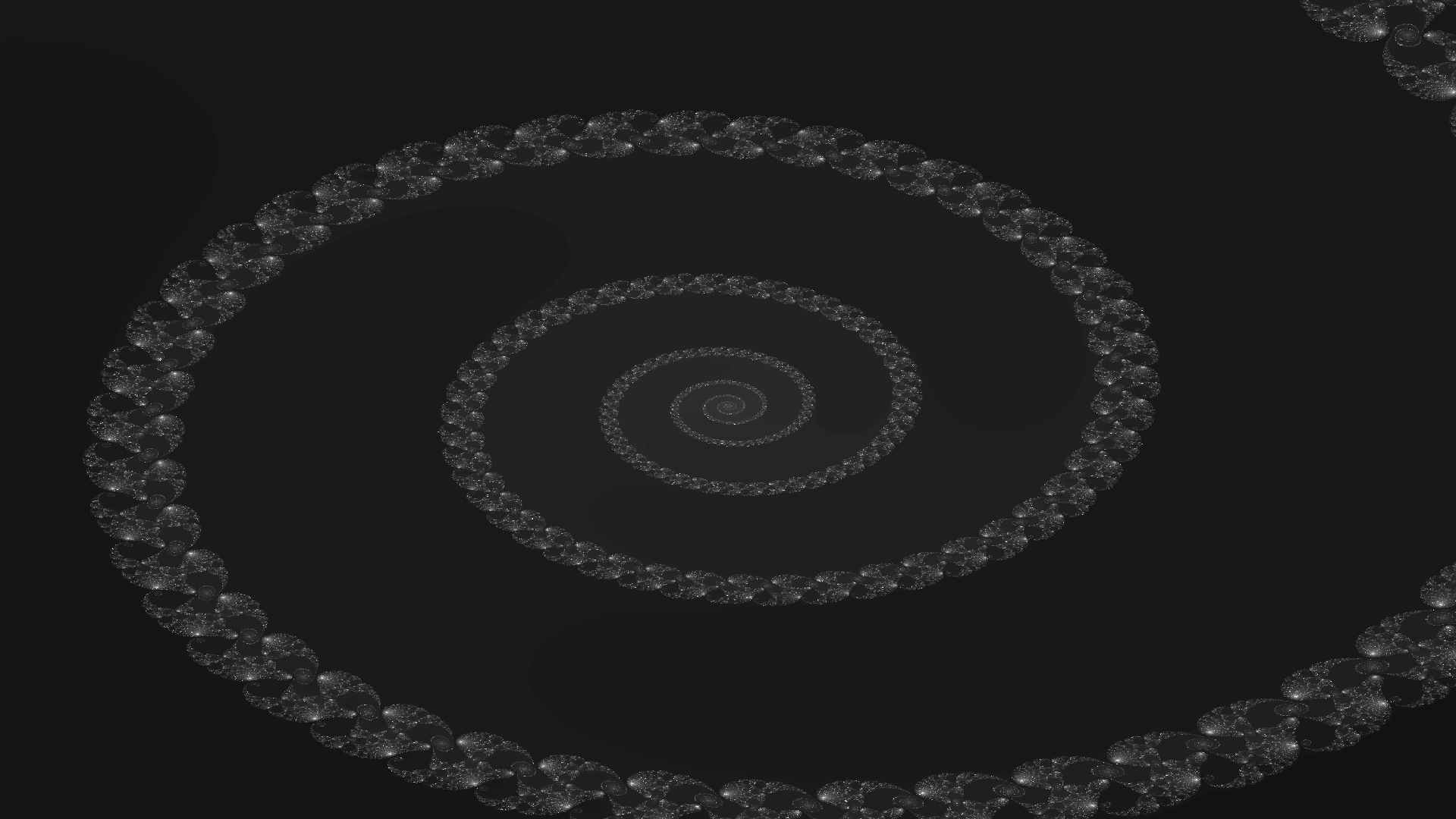
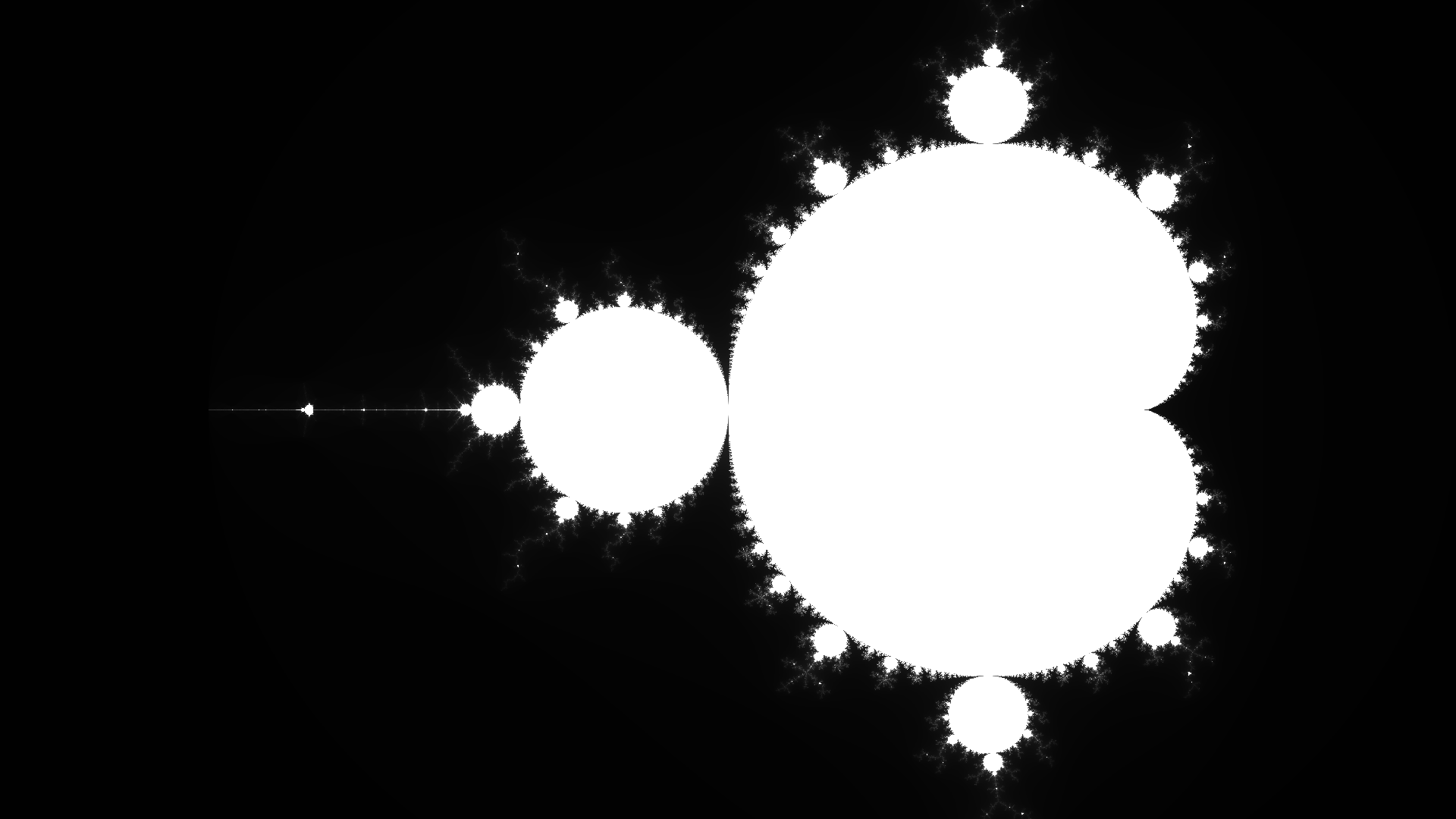
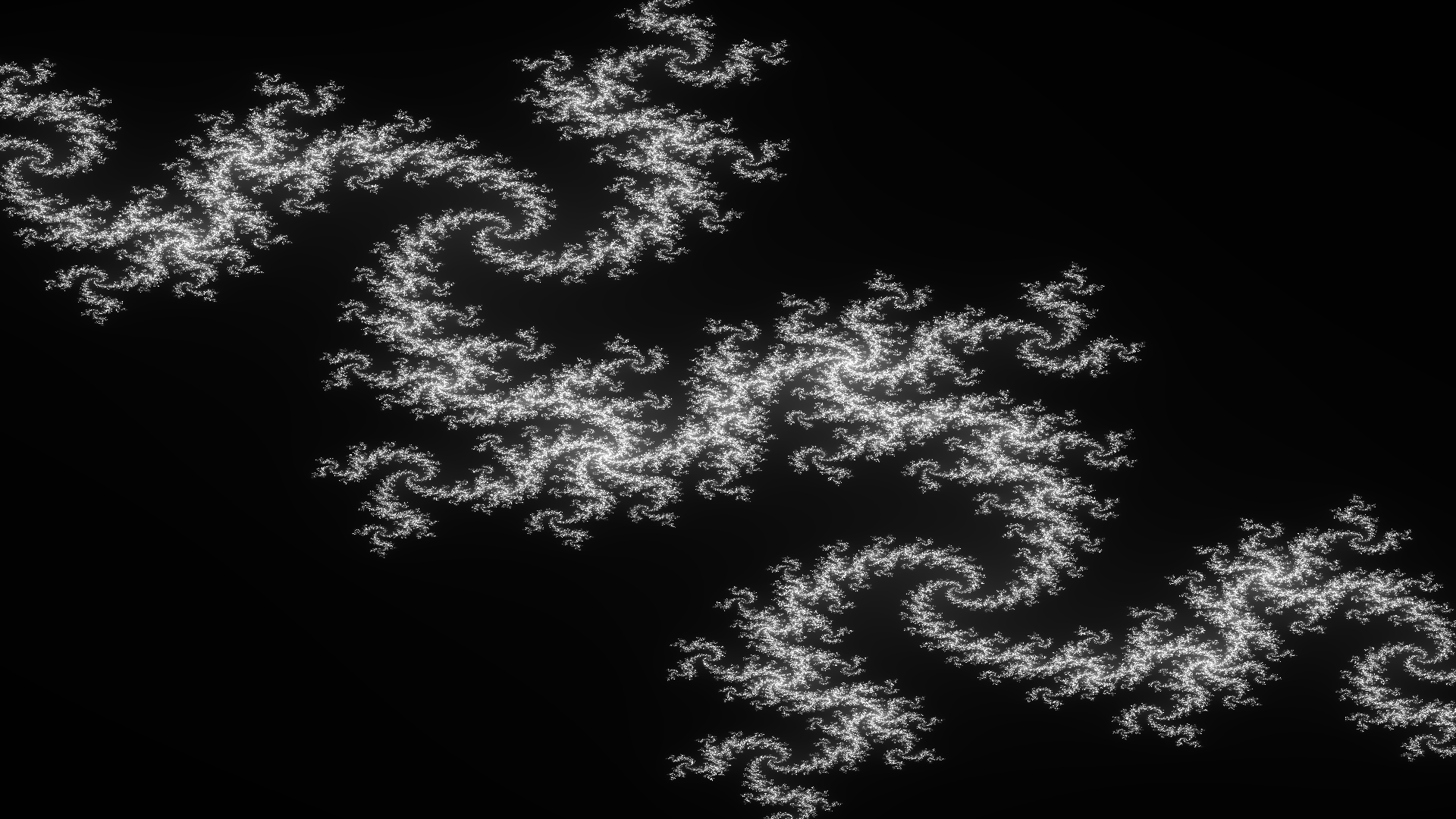
Links:
Description
The idea behind this small challenge was to create a mastermind game for a user to play. The original concept was to allow for just textual inputs, and so have the game as a terminal based one. However, I found that the challenge was finished fairly quickly, so I decided to use this challenge as a way of recalling java swing and to learn javaFX.
The swing game was not too hard to create as I already knew all of the components and how they interacted with each other from when I was making mwindow. This meant that all I needed to do was to plan out the games UI layout. Once the layout draft had been made and I had a rough idea of how to implement it, I started to write the code for it. As far as actually writing the code for the swing application, I didn't have any real issues with it.
The javaFX version of the game however was more challenging to me as it was a new set of classes and window lifecycle to get used to. Luckily I have had a little bit of experience with android UI development and so getting used to the javaFX packages wasn't too much of a stretch. The Scene Builder tool allows for easy creation of FXML files, this made designing the UI much easier. Once I had gotten my head around how the javaFX packages work, I found them to be more useful than its java swing counterpart. Not to mention the fact that the basic look and feel for the javaFX components is much better.
Screenshots:
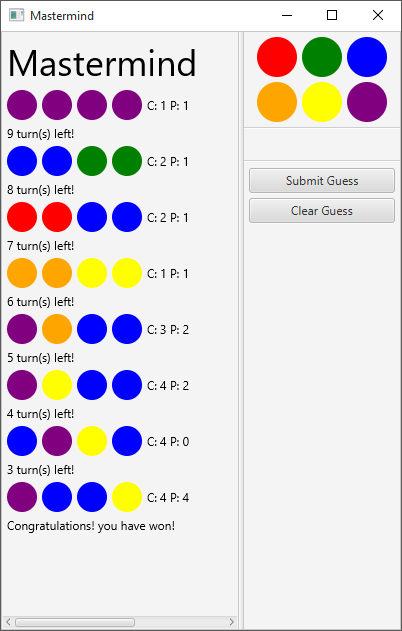
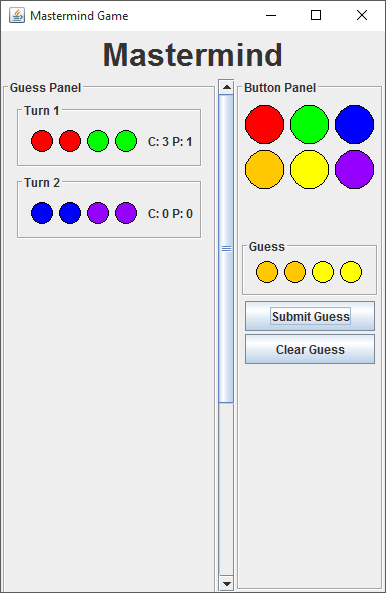
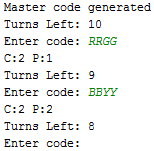
Links:
Description
This challenge was picked from the r/dailyprogrammer subreddit. The challenge posed was to make a copy of the fallout hacking mini-game by pulling words from a given dictionary. The mini-game is a similar game to mastermind where the player has to guess a word and the game will tell them how many letters in the guessed word is in the correct position.
Examples
Output
What difficulty of game do you want? [1/2/3]
2
WAFTING
OVERRUN
SUPPERS
BLUNGES
OUTTROT
ECTOPIC
FUSSPOT
HOISTER
BURLIER
DALTONS
LUGSAIL
Enter your guess: wafting
7 letters correct
Congratulations you have guessed correctly!